Pytorchでテキスト分類チュートリアル実施
またPytorchのチュートリアルを実践してみます。今回は自然言語処理でテキスト分類を試してみました。
参考はこちらのオフィシャルサイトからですね。
https://pytorch.org/tutorials/beginner/text_sentiment_ngrams_tutorial.html
自然言語処理はまた専用の処理がたくさんあって勉強することが多いですね。
使うデータセット
ここではAG_NEWSというデータセットでニュースを4種類に分類するチュートリアルになってますね。
AG_NEWSの公式サイトはこちらみたいですね。2004年からコツコツ集めたニュースコーパスということでしょうか。
http://www.di.unipi.it/~gulli/AG_corpus_of_news_articles.html
train.csv
test.csv
classes.txt
readme.txt
の4つのファイルからなるシンプルなデータセットですね。
train.csv,test.csvは1列目が正解ラベル分類(1から4)、2列目がニュース本文のデータです。
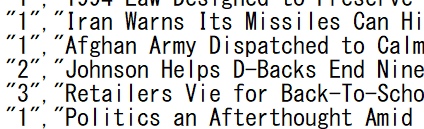
分類コードはこれですね(classes.txt)。
1 : "World",
2 : "Sports",
3 : "Business",
4 : "Sci/Tec"
モデル
チュートリアルのモデルは EmbeddingBag-Linear(全結合)の単純なモデルですね。自然言語処理なので文字のベクトル化のためにEmbeddingBag層が入っているのが自然言語処理らしいところですね。
モデルは学習・推論で使うので、ここでは別ファイルでmodel.pyとして作成してみます。
#model.py
import torch.nn as nn
import torch.nn.functional as F
class TextSentiment(nn.Module):
def __init__(self, vocab_size, embed_dim, num_class):
super().__init__()
self.embedding = nn.EmbeddingBag(vocab_size, embed_dim, sparse=True)
self.fc = nn.Linear(embed_dim, num_class)
self.init_weights()
def init_weights(self):
initrange = 0.5
self.embedding.weight.data.uniform_(-initrange, initrange)
self.fc.weight.data.uniform_(-initrange, initrange)
self.fc.bias.data.zero_()
def forward(self, text, offsets):
embedded = self.embedding(text, offsets)
return self.fc(embedded)
EmbeddingBag と「Bag」がついてますので、「Bag of Word(BoW)」なのかなと予想できますね。単語の出現回数を数える手法ですね。日本語ですと追加で形態素解析で単語にする作業が必要そうですが、今回は英文のままなので、形態素解析は不要ですね。
訓練実施
コードをまとめるとこちらですね。最後に学習済みモデルファイルをtext.pthとして保存します。処理の流れは通常のPytorchの学習と同じですね。
#train.py
import torch
import torchtext
from torchtext.datasets import text_classification
from model import TextSentiment
NGRAMS = 2
import os
if not os.path.isdir('./.data'):
os.mkdir('./.data')
train_dataset, test_dataset = text_classification.DATASETS['AG_NEWS'](
root='./.data', ngrams=NGRAMS, vocab=None)
BATCH_SIZE = 16
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
VOCAB_SIZE = len(train_dataset.get_vocab())
EMBED_DIM = 32
NUN_CLASS = len(train_dataset.get_labels())
model = TextSentiment(VOCAB_SIZE, EMBED_DIM, NUN_CLASS).to(device)
def generate_batch(batch):
label = torch.tensor([entry[0] for entry in batch])
text = [entry[1] for entry in batch]
offsets = [0] + [len(entry) for entry in text]
# torch.Tensor.cumsum returns the cumulative sum
# of elements in the dimension dim.
# torch.Tensor([1.0, 2.0, 3.0]).cumsum(dim=0)
offsets = torch.tensor(offsets[:-1]).cumsum(dim=0)
text = torch.cat(text)
return text, offsets, label
from torch.utils.data import DataLoader
def train_func(sub_train_):
# Train the model
train_loss = 0
train_acc = 0
data = DataLoader(sub_train_, batch_size=BATCH_SIZE, shuffle=True,
collate_fn=generate_batch)
for i, (text, offsets, cls) in enumerate(data):
optimizer.zero_grad()
text, offsets, cls = text.to(device), offsets.to(device), cls.to(device)
output = model(text, offsets)
loss = criterion(output, cls)
train_loss += loss.item()
loss.backward()
optimizer.step()
train_acc += (output.argmax(1) == cls).sum().item()
# Adjust the learning rate
scheduler.step()
return train_loss / len(sub_train_), train_acc / len(sub_train_)
def test(data_):
loss = 0
acc = 0
data = DataLoader(data_, batch_size=BATCH_SIZE, collate_fn=generate_batch)
for text, offsets, cls in data:
text, offsets, cls = text.to(device), offsets.to(device), cls.to(device)
with torch.no_grad():
output = model(text, offsets)
loss = criterion(output, cls)
loss += loss.item()
acc += (output.argmax(1) == cls).sum().item()
return loss / len(data_), acc / len(data_)
import time
from torch.utils.data.dataset import random_split
N_EPOCHS = 5
min_valid_loss = float('inf')
criterion = torch.nn.CrossEntropyLoss().to(device)
optimizer = torch.optim.SGD(model.parameters(), lr=4.0)
scheduler = torch.optim.lr_scheduler.StepLR(optimizer, 1, gamma=0.9)
train_len = int(len(train_dataset) * 0.95)
sub_train_, sub_valid_ = \
random_split(train_dataset, [train_len, len(train_dataset) - train_len])
for epoch in range(N_EPOCHS):
start_time = time.time()
train_loss, train_acc = train_func(sub_train_)
valid_loss, valid_acc = test(sub_valid_)
secs = int(time.time() - start_time)
mins = secs / 60
secs = secs % 60
print('Epoch: %d' %(epoch + 1), " | time in %d minutes, %d seconds" %(mins, secs))
print(f'\tLoss: {train_loss:.4f}(train)\t|\tAcc: {train_acc * 100:.1f}%(train)')
print(f'\tLoss: {valid_loss:.4f}(valid)\t|\tAcc: {valid_acc * 100:.1f}%(valid)')
print('Checking the results of test dataset...')
test_loss, test_acc = test(test_dataset)
print(f'\tLoss: {test_loss:.4f}(test)\t|\tAcc: {test_acc * 100:.1f}%(test)')
torch.save(model.state_dict(), './text.pth')
学習させるデータ変数はtext, offsets, clsの3つがありますが、こちらはバッチサイズ16の学習データをまとめたものになってますね。
#text 16個のニュースをまとめてベクトル化した長さ1374の配列(配列長さはバッチによる)
tensor([ 30, 17, 10, ..., 849600, 557690, 1818])
#offset textの配列で16個ニュースのスタート位置。例えば147から217までは2番目のニュース
tensor([ 0, 147, 218, 261, 326, 423, 484, 547, 622, 691, 870, 961,
1032, 1113, 1200, 1271])
#cls 正解ラベル。16個。
([1, 2, 1, 2, 0, 3, 2, 2, 1, 3, 3, 0, 2, 2, 1, 2])
実行はこちらです。
$ python train.py
出力の後半はこんなですね。テストの正解率が89%ですね。
Epoch: 5 | time in 1 minutes, 58 seconds
Loss: 0.0023(train) | Acc: 99.0%(train)
Loss: 0.0000(valid) | Acc: 91.1%(valid)
Checking the results of test dataset...
Loss: 0.0003(test) | Acc: 89.0%(test)
モデルファイルを呼び出して推論
訓練で作成したtext.pthをロードして推論を別途実行してみます。ニュースはVOANEWSから適当に拝借してみました。
#predict.py
import re
from torchtext.data.utils import ngrams_iterator
from torchtext.data.utils import get_tokenizer
from model import TextSentiment
from torchtext.datasets import text_classification
import torch
ag_news_label = {1 : "World",
2 : "Sports",
3 : "Business",
4 : "Sci/Tec"}
def predict(text, model, vocab, ngrams):
tokenizer = get_tokenizer("basic_english")
with torch.no_grad():
text = torch.tensor([vocab[token]
for token in ngrams_iterator(tokenizer(text), ngrams)])
output = model(text, torch.tensor([0]))
return output.argmax(1).item() + 1
ex_text_str="WASHINGTON - U.S. Treasury Secretary Steven Mnuchin says a \
new coronavirus economic aid package being unveiled Monday would \
pay the country’s 16 million unemployed workers \
70% of their one-time salaries, but tough negotiations lie \
ahead with opposition Democrats over the scope of more assistance \
to offset the severe economic effects of the pandemic. "
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
NGRAMS = 2
train_dataset, test_dataset = text_classification.DATASETS['AG_NEWS'](
root='./.data', ngrams=NGRAMS, vocab=None)
vocab = train_dataset.get_vocab()
VOCAB_SIZE = len(train_dataset.get_vocab())
EMBED_DIM = 32
NUN_CLASS = len(train_dataset.get_labels())
model = TextSentiment(VOCAB_SIZE, EMBED_DIM, NUN_CLASS).to(device)
model.load_state_dict(torch.load('text.pth'))
print("This is a %s news" %ag_news_label[predict(ex_text_str, model, vocab, 2)])
ニュース元。
https://www.voanews.com/covid-19-pandemic/white-house-looks-cover-70-jobless-workers-previous-wages
ちなみにこのニュースをベクトル化するとこんなデータ構造になってますね。
#ex_text_str->text
tensor([ 197, 16, 64, 2, 10, 2, 2717, 1008,
9529, 0, 112, 6, 27, 0, 489, 886,
2897, 376, 1475, 74, 128, 477, 3, 0,
1007, 110, 84518, 535, 0, 7, 50, 12129,
20777, 4, 55, 1798, 2054, 16509, 473, 20,
998, 3942, 48, 3, 33868, 7, 56, 13577,
5, 4717, 3, 6676, 489, 5496, 7, 3,
41271, 2, 2088, 699, 65, 73, 68, 7741,
10497, 0, 0, 0, 5609, 151, 0, 0,
745259, 313474, 0, 0, 289482, 960996, 38338, 35035,
0, 0, 171420, 0, 0, 0, 0, 925,
1216886, 0, 275847, 118, 0, 465748, 0, 130972,
6178, 105310, 0, 0, 204, 84084, 56818, 4328,
962667, 58292, 21334, 37793, 285161, 442662, 354022, 9588,
29, 1212014, 199128])
またoffsetはニュースが一つだけですので、torch.tensor([0]) が使われてますね。
これで実行です。
$ python predict.py
判定は 「3 : “Business”」 ですね。あってそうです。ちなみに結果のoutputの中身はone-hot形式になってますね。 3番目が一番大きいですね。
tensor([[ 3.1121, -10.2911, 5.0545, 2.1624]])
また訓練では隠れてましたが、推論では登場しているvocabが肝のような気がしますね。
https://torchtext.readthedocs.io/en/latest/vocab.html
vocabの中をちょっと覗いてみます。
#vocab.freqs
Counter({'.': 225971, 'the': 203843, ',': 165685, 'to': 119205, 'a': 110153, 'of': 97909, 'in': 95488, 'and': 68872, 's': 61724, 'on': 56529, 'for': 50186, '#39': 44316, '(': 41106, ')': 40787, '-': 39206, "'": 32235, '#39 s': 31220, 'that': 27993, 'with': 26682, 'as': 25176, 'at': 24914, "' s": 22313, 'is': 22001, 'its': 21925, 'in the': 21343,
#vocab.stoi
{'<unk>': 0, '<pad>': 1, '.': 2, 'the': 3, ',': 4, 'to': 5, 'a': 6, 'of': 7, 'in': 8, 'and': 9, 's': 10, 'on': 11, 'for': 12, '#39': 13, '(': 14, ')': 15, '-': 16, "'": 17, '#39 s': 18, 'that': 19, 'with': 20, 'as': 21, 'at': 22, "' s": 23, 'is': 24, 'its': 25, 'in the': 26,
vocab.freqsを見ると単語が多い順に並んでいるのがわかりますね。”.”(ピリオド)、”the”や前置詞(to,of,in)が多いのは予想通りですかね。
vocab.stoiをみると出現回数の多い単語順にインデックス化しているのがわかりますね(除く<unk>と<pad>)。
ただNGRAMS=2にしているので単語2つになりそうですが、単語1つと2つが処理されてるみたいですね。そういうものなんですかね。
https://pytorch.org/text/data_utils.html
このあたりデータの処理をみると実際にはこの順序で文字が処理されています。ちょっと冗長ですが、のせときます。始めは一単語、そのあと二単語処理されてます。
washington ->197に。
- ->16に。
u ->64に。
. ->2に。
s ->10に。
. ->2に。
treasury
secretary
steven
mnuchin
says
a
new
coronavirus
economic
aid
package
being
unveiled
monday
would
pay
the
country’s
16
million
unemployed
workers
70%
of
their
one-time
salaries
,
but
tough
negotiations
lie
ahead
with
opposition
democrats
over
the
scope
of
more
assistance
to
offset
the
severe
economic
effects
of
the
pandemic
.
washington -
- u
u .
. s
s .
. treasury
treasury secretary
secretary steven
steven mnuchin
mnuchin says
says a
a new
new coronavirus
coronavirus economic
economic aid
aid package
package being
being unveiled
unveiled monday
monday would
would pay
pay the
the country’s
country’s 16
16 million
million unemployed
unemployed workers
workers 70%
70% of
of their
their one-time
one-time salaries
salaries ,
, but
but tough
tough negotiations
negotiations lie
lie ahead
ahead with
with opposition
opposition democrats
democrats over
over the
the scope
scope of
of more
more assistance
assistance to
to offset
offset the
the severe
severe economic
economic effects
effects of
of the
the pandemic
pandemic .
まとめ
オフィシャルサイトの解説を読んでもさっぱりわかりませんが、実際のデータの中身を追っていくと多少わかったような気になりますね。。